The Trojan
The Trojan is a top down, horror, action game that I developed alongside one other developer. I designed and implemented the character controller, object interactions, the enemies, the player's inventory, the gun system, and all of the other things that the player interacts with throughout a playthrough of the game. The Trojan was my first full gameplay experience made in the Unreal Engine 5, and programmed with a combination of C++ for the player character, inventory, parts of the enemies, and Blueprints to handle enemy AI chasing, UI and VFX, and doors.
The Player
The player character has a few things that the player is able to constantly interact and use.
​
Firstly, the player constantly looks at the mouse cursor during play, and this was done by getting the mouse's position and direction from the player, projecting those two variables from the screen into the game world, casting a ray to find the point at which the mouse currently is, and finally setting the player's rotation to match where the mouse is in relation to them.
​
Secondly, the player has an inventory that they can access. This inventory is handled by an actor component that's attached to the player which has an array of objects that can be added to or removed from, and updates the UI.
​
Thirdly, the player is able to interact with various objects that they find around the play space by right clicking on them to: Read Messages, Add Items to Inventory, Add Ammo, and Heal.

Code for looking at the cursor
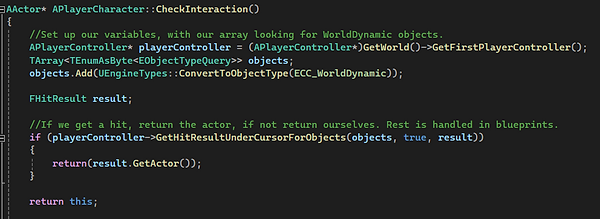
Code for interacting with items based on the cursor, the interaction is handled in blueprints and the items own code

Code that all Interactable Objects can have in order to be added to the inventory of the player character.
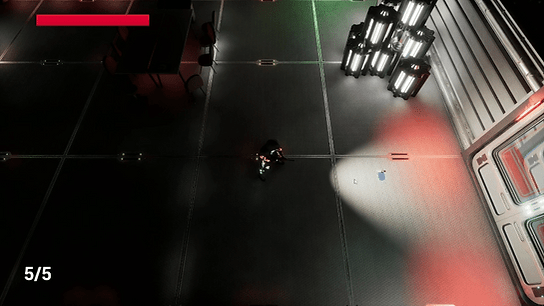
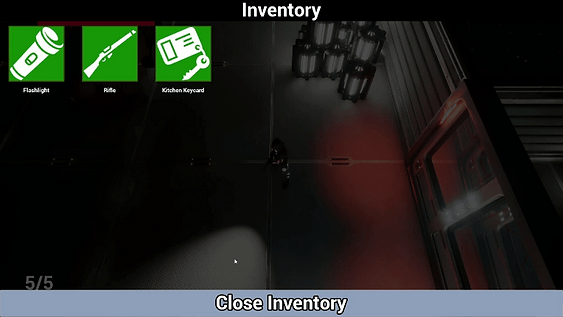
The Inventory
We knew from the get go that we needed the player to have an inventory. This was to help them keep track of the various objects they would pick up along the course of playing the game, as well as a guideline for how to build an inventory for future projects.
​
In The Trojan, the inventory object is an actor component that we add onto the player character. This component houses all of the Inventory Objects in the game in an array of Interactable Objects, and helps to update the display when an item is added or removed from the array.
​
Each Inventory object is a custom class that was built that is comprised of: A Picture that is shown when opening the inventory, A Name that is shown when picking up the item and when seeing it in the inventory, and A Description that is shown when hovering the object with your cursor in the inventory.
​
In addition there is a UI element to alert the player to what they have just picked up or interacted with. This happens when the player: Interacts with a door, picks up an item, uses one of the healing items, picks up ammo, or uses an item to unlock something.
​
Finally they each have two functions, one for use in C++ and the other for use in Blueprints. The C++ is most commonly used to add the item to the player's inventory component, and change values of the player (such as when the gun is picked up). The blueprint function is used to take care of hiding the object in the scene so the player can't accidentally get two flashlights.
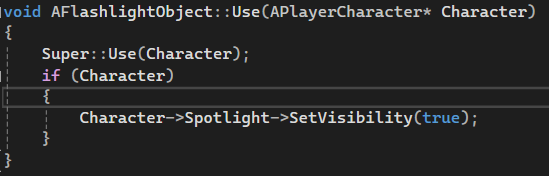
Code to show the specific use case of an Interactable Object. In this case, switching the spotlight to being on to mimic a flashlight for the player.
The Enemy
Enemies in The Trojan are designed to be simple, as they're there to provide a momentary amount of scariness when they first come into contact with the player, and give a brief round of combat.
​
For this, the enemy uses a Nav Mesh to move around the level and chase down the player character. When the enemy gets close enough to the player, they stop and attack the player to deal damage, then resume chasing.
​
The start of the chase happens either when the player comes into line of sight of the enemy, or when the player comes too close to the enemy.
​
The enemy also needs to remain in the level, but motionless and unable to attack, when the enemy's health reached 0. So once the health reaches 0, the ability to attack is removed, the ability to move is removed, and the enemy's collision is set to a no collision mode while playing a death noise.
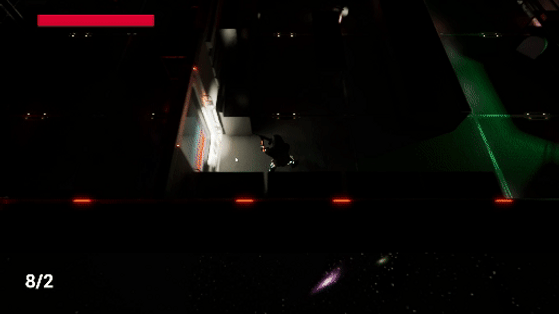
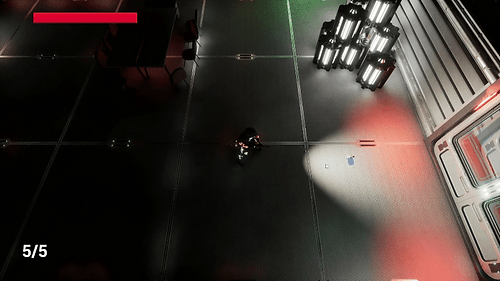
The pickup UI's delegate is notified by the player that an object has been picked up, and displays the appropriate text. The Inventory component's delegate is notified that something has been added, and it updates the inventory's display.

Blueprints showing how inventory is updated. The delegate gets called and then uses the Initialize Inventory function which does a process of: Clearing the current Inventory UI, looping through our current inventory, creating an Inventory Item Widget for each item found in our Items array, and then adding that widget to our Wrap Box which is displayed to the player.
The UI
The UI within The Trojan is made to be simple as there's very few things that will appear on the screen at any given point, and very few things that the player needs to keep track of as they move throughout the level.
​
The health, ammo, pickup notifications, and inventory are all handled through a delegate system. Where delegates are created in C++ on either the player, the enemy, or the inventory items, and then are broadcasted to help update the UI. These updates happen for: shooting the gun, picking up objects, taking or healing damage, and will also trigger animation montages.
​
The reason I went with a delegate system for the game is that it was simply the easiest and most accessible way to have my C++ code talk directly to widget blueprints, and would allow me to make sure the player is getting accurate showings of what is happening to their character.
​
In addition, the delegates were not used for everything. For the messages that appear in game, we used a variation of the items that go into the inventory. This allowed me to not have to reprogram the ability to interact with the objects themselves, and still add on the functionality of adding the messages to the player viewport which allows them to get vital information about the story of The Trojan.